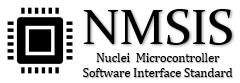 |
NMSIS-Core
Version 1.3.1
NMSIS-Core support for Nuclei processor-based devices
|
18 #ifndef __CORE_FEATURE_CACHE_H__
19 #define __CORE_FEATURE_CACHE_H__
40 #include "core_feature_base.h"
43 #if defined(__CCM_PRESENT) && (__CCM_PRESENT == 1)
106 #if __riscv_xlen == 32
107 #define CCM_SUEN_SUEN_Msk (0xFFFFFFFFUL)
109 #define CCM_SUEN_SUEN_Msk (0xFFFFFFFFFFFFFFFFUL)
160 #if defined(__ICACHE_PRESENT) && (__ICACHE_PRESENT == 1)
246 #if defined(__CCM_PRESENT) && (__CCM_PRESENT == 1)
264 info->setperway = (1 << csr_ccfg.
b.
set) << 3;
265 info->ways = (1 + csr_ccfg.
b.
way);
266 if (csr_ccfg.
b.
lsize == 0) {
269 info->linesize = (1 << (csr_ccfg.
b.
lsize - 1)) << 3;
271 info->size = info->setperway * info->ways * info->linesize;
307 for (i = 0; i < cnt; i++) {
345 for (i = 0; i < cnt; i++) {
383 for (i = 0; i < cnt; i++) {
424 for (i = 0; i < cnt; i++) {
470 for (i = 0; i < cnt; i++) {
516 for (i = 0; i < cnt; i++) {
558 for (i = 0; i < cnt; i++) {
595 for (i = 0; i < cnt; i++) {
632 for (i = 0; i < cnt; i++) {
683 #if defined(__DCACHE_PRESENT) && (__DCACHE_PRESENT == 1)
768 #if defined(__CCM_PRESENT) && (__CCM_PRESENT == 1)
786 info->setperway = (1 << csr_ccfg.
b.
set) << 3;
787 info->ways = (1 + csr_ccfg.
b.
way);
788 if (csr_ccfg.
b.
lsize == 0) {
791 info->linesize = (1 << (csr_ccfg.
b.
lsize - 1)) << 3;
793 info->size = info->setperway * info->ways * info->linesize;
829 for (i = 0; i < cnt; i++) {
867 for (i = 0; i < cnt; i++) {
905 for (i = 0; i < cnt; i++) {
942 for (i = 0; i < cnt; i++) {
979 for (i = 0; i < cnt; i++) {
1016 for (i = 0; i < cnt; i++) {
1053 for (i = 0; i < cnt; i++) {
1090 for (i = 0; i < cnt; i++) {
1127 for (i = 0; i < cnt; i++) {
1168 for (i = 0; i < cnt; i++) {
1214 for (i = 0; i < cnt; i++) {
1260 for (i = 0; i < cnt; i++) {
1302 for (i = 0; i < cnt; i++) {
1339 for (i = 0; i < cnt; i++) {
1376 for (i = 0; i < cnt; i++) {
rv_csr_t d
Type used for csr data access.
__STATIC_FORCEINLINE int32_t DCachePresent(void)
Check DCache Unit Present or Not.
__STATIC_FORCEINLINE void DisableICacheECC(void)
Disable ICache ECC.
__STATIC_FORCEINLINE void DisableDCache(void)
Disable DCache.
__STATIC_FORCEINLINE void SInvalDCacheLines(unsigned long addr, unsigned long cnt)
Invalidate several D-Cache lines specified by address in S-Mode.
__STATIC_FORCEINLINE void UUnlockICacheLines(unsigned long addr, unsigned long cnt)
Unlock several I-Cache lines specified by address in U-Mode.
__STATIC_FORCEINLINE void MInvalDCacheLine(unsigned long addr)
Invalidate one D-Cache line specified by address in M-Mode.
__STATIC_FORCEINLINE void SFlushDCacheLines(unsigned long addr, unsigned long cnt)
Flush several D-Cache lines specified by address in S-Mode.
__STATIC_FORCEINLINE void SFlushInvalDCacheLines(unsigned long addr, unsigned long cnt)
Flush and invalidate several D-Cache lines specified by address in S-Mode.
__STATIC_FORCEINLINE unsigned long ULockDCacheLines(unsigned long addr, unsigned long cnt)
Lock several D-Cache lines specified by address in U-Mode.
__STATIC_FORCEINLINE void MInvalICacheLines(unsigned long addr, unsigned long cnt)
Invalidate several I-Cache lines specified by address in M-Mode.
__STATIC_FORCEINLINE unsigned long ULockDCacheLine(unsigned long addr)
Lock one D-Cache line specified by address in U-Mode.
__STATIC_FORCEINLINE unsigned long MLockDCacheLine(unsigned long addr)
Lock one D-Cache line specified by address in M-Mode.
__STATIC_FORCEINLINE void EnableSUCCM(void)
Enable CCM operation in Supervisor/User Mode.
@ CCM_IC_INVAL_ALL
Unlock and invalidate all the I-Cache lines.
rv_csr_t lsize
I-Cache line size.
__STATIC_FORCEINLINE void UInvalDCache(void)
Invalidate all D-Cache lines in U-Mode.
__STATIC_FORCEINLINE unsigned long MLockICacheLine(unsigned long addr)
Lock one I-Cache line specified by address in M-Mode.
#define CSR_CCM_MBEGINADDR
#define __RV_CSR_CLEAR(csr, val)
CSR operation Macro for csrc instruction.
__STATIC_FORCEINLINE void UFlushInvalDCache(void)
Flush and invalidate all D-Cache lines in U-Mode.
__STATIC_FORCEINLINE unsigned long ULockICacheLine(unsigned long addr)
Lock one I-Cache line specified by address in U-Mode.
@ CCM_DC_LOCK
Lock the specific D-Cache line specified by CSR CCM_XBEGINADDR.
@ CCM_OP_REFILL_BUS_ERR
Refill has Bus Error.
Union type to access MICFG_INFO CSR register.
__STATIC_FORCEINLINE void MFlushInvalDCache(void)
Flush and invalidate all D-Cache lines in M-Mode.
__STATIC_FORCEINLINE void SUnlockICacheLine(unsigned long addr)
Unlock one I-Cache line specified by address in S-Mode.
__STATIC_FORCEINLINE void UInvalDCacheLine(unsigned long addr)
Invalidate one D-Cache line specified by address in U-Mode.
__STATIC_FORCEINLINE void SUnlockDCacheLines(unsigned long addr, unsigned long cnt)
Unlock several D-Cache lines specified by address in S-Mode.
__STATIC_FORCEINLINE void SFlushDCacheLine(unsigned long addr)
Flush one D-Cache line specified by address in S-Mode.
__STATIC_FORCEINLINE void UUnlockICacheLine(unsigned long addr)
Unlock one I-Cache line specified by address in U-Mode.
Union type to access MDCFG_INFO CSR register.
rv_csr_t d
Type used for csr data access.
__STATIC_FORCEINLINE void SFlushInvalDCacheLine(unsigned long addr)
Flush and invalidate one D-Cache line specified by address in S-Mode.
__STATIC_FORCEINLINE void UFlushDCache(void)
Flush all D-Cache lines in U-Mode.
CCM_CMD
Cache CCM Command Types.
__STATIC_FORCEINLINE void MFlushDCacheLine(unsigned long addr)
Flush one D-Cache line specified by address in M-Mode.
@ CCM_OP_ECC_ERR
Deprecated, ECC Error, this error code is removed in later Nuclei CCM RTL design, please don't use it...
#define __RV_CSR_WRITE(csr, val)
CSR operation Macro for csrw instruction.
__STATIC_FORCEINLINE void UFlushInvalDCacheLine(unsigned long addr)
Flush and invalidate one D-Cache line specified by address in U-Mode.
uint32_t setperway
Cache set per way.
__STATIC_FORCEINLINE void DisableDCacheECC(void)
Disable DCache ECC.
__STATIC_FORCEINLINE void SInvalDCacheLine(unsigned long addr)
Invalidate one D-Cache line specified by address in S-Mode.
__STATIC_FORCEINLINE void FlushPipeCCM(void)
Flush pipeline after CCM operation.
__STATIC_FORCEINLINE void UInvalICacheLines(unsigned long addr, unsigned long cnt)
Invalidate several I-Cache lines specified by address in U-Mode.
uint32_t size
Cache total size in bytes.
__STATIC_FORCEINLINE void MUnlockICacheLine(unsigned long addr)
Unlock one I-Cache line specified by address in M-Mode.
__STATIC_FORCEINLINE int32_t GetICacheInfo(CacheInfo_Type *info)
Get I-Cache Information.
__STATIC_FORCEINLINE void EnableDCacheECC(void)
Enable DCache ECC.
#define CCM_SUEN_SUEN_Msk
CSR CCM_SUEN: SUEN Mask.
__STATIC_FORCEINLINE void SUnlockDCacheLine(unsigned long addr)
Unlock one D-Cache line specified by address in S-Mode.
@ CCM_DC_WBINVAL_ALL
Unlock and flush and invalidate all the valid and dirty D-Cache lines.
__STATIC_FORCEINLINE void UUnlockDCacheLine(unsigned long addr)
Unlock one D-Cache line specified by address in U-Mode.
__STATIC_FORCEINLINE unsigned long ULockICacheLines(unsigned long addr, unsigned long cnt)
Lock several I-Cache lines specified by address in U-Mode.
__STATIC_FORCEINLINE void EnableDCache(void)
Enable DCache.
@ CCM_OP_SUCCESS
Lock Succeed.
__STATIC_FORCEINLINE void UInvalICache(void)
Invalidate all I-Cache lines in U-Mode.
__STATIC_FORCEINLINE void SFlushDCache(void)
Flush all D-Cache lines in S-Mode.
__STATIC_FORCEINLINE void MInvalDCache(void)
Invalidate all D-Cache lines in M-Mode.
__STATIC_FORCEINLINE void MFlushInvalDCacheLines(unsigned long addr, unsigned long cnt)
Flush and invalidate several D-Cache lines specified by address in M-Mode.
__STATIC_FORCEINLINE unsigned long SLockICacheLine(unsigned long addr)
Lock one I-Cache line specified by address in S-Mode.
struct CSR_MDCFGINFO_Type::@14 b
Structure used for bit access.
@ CCM_IC_INVAL
Unlock and invalidate I-Cache line specified by CSR CCM_XBEGINADDR.
@ CCM_DC_UNLOCK
Unlock the specific D-Cache line specified by CSR CCM_XBEGINADDR.
__STATIC_FORCEINLINE void UFlushInvalDCacheLines(unsigned long addr, unsigned long cnt)
Flush and invalidate several D-Cache lines specified by address in U-Mode.
__STATIC_FORCEINLINE void SFlushInvalDCache(void)
Flush and invalidate all D-Cache lines in S-Mode.
uint32_t linesize
Cache Line size in bytes.
rv_csr_t set
I-Cache sets per way.
struct CSR_MICFGINFO_Type::@13 b
Structure used for bit access.
@ CCM_DC_WB_ALL
Flush all the valid and dirty D-Cache lines.
__STATIC_FORCEINLINE void DisableSUCCM(void)
Disable CCM operation in Supervisor/User Mode.
__STATIC_FORCEINLINE void MFlushDCacheLines(unsigned long addr, unsigned long cnt)
Flush several D-Cache lines specified by address in M-Mode.
__STATIC_FORCEINLINE void MUnlockICacheLines(unsigned long addr, unsigned long cnt)
Unlock several I-Cache lines specified by address in M-Mode.
#define __STATIC_FORCEINLINE
Define a static function that should be always inlined by the compiler.
__STATIC_FORCEINLINE void MInvalICache(void)
Invalidate all I-Cache lines in M-Mode.
__STATIC_FORCEINLINE void UFlushDCacheLines(unsigned long addr, unsigned long cnt)
Flush several D-Cache lines specified by address in U-Mode.
@ CCM_OP_PERM_CHECK_ERR
PMP/sPMP/Page-Table X(I-Cache)/R(D-Cache) permission check failed, or belong to Device/Non-Cacheable ...
__STATIC_FORCEINLINE void MFlushInvalDCacheLine(unsigned long addr)
Flush and invalidate one D-Cache line specified by address in M-Mode.
__STATIC_FORCEINLINE unsigned long SLockICacheLines(unsigned long addr, unsigned long cnt)
Lock several I-Cache lines specified by address in S-Mode.
#define MCACHE_CTL_DC_ECC_EN
#define CSR_CCM_SBEGINADDR
__STATIC_FORCEINLINE void MInvalICacheLine(unsigned long addr)
Invalidate one I-Cache line specified by address in M-Mode.
__STATIC_FORCEINLINE void UInvalICacheLine(unsigned long addr)
Invalidate one I-Cache line specified by address in U-Mode.
__STATIC_FORCEINLINE unsigned long SLockDCacheLines(unsigned long addr, unsigned long cnt)
Lock several D-Cache lines specified by address in S-Mode.
__STATIC_FORCEINLINE void MInvalDCacheLines(unsigned long addr, unsigned long cnt)
Invalidate several D-Cache lines specified by address in M-Mode.
rv_csr_t set
D-Cache sets per way.
#define __RV_CSR_READ(csr)
CSR operation Macro for csrr instruction.
__STATIC_FORCEINLINE void SInvalICache(void)
Invalidate all I-Cache lines in S-Mode.
__STATIC_FORCEINLINE unsigned long MLockDCacheLines(unsigned long addr, unsigned long cnt)
Lock several D-Cache lines specified by address in M-Mode.
CCM_OP_FINFO
Cache CCM Operation Fail Info.
@ CCM_DC_WB
Flush the specific D-Cache line specified by CSR CCM_XBEGINADDR.
__STATIC_FORCEINLINE void MFlushDCache(void)
Flush all D-Cache lines in M-Mode.
__STATIC_FORCEINLINE void EnableICacheECC(void)
Enable ICache ECC.
#define __RV_CSR_SET(csr, val)
CSR operation Macro for csrs instruction.
__STATIC_FORCEINLINE void UUnlockDCacheLines(unsigned long addr, unsigned long cnt)
Unlock several D-Cache lines specified by address in U-Mode.
__STATIC_FORCEINLINE void DisableICache(void)
Disable ICache.
@ CCM_DC_INVAL_ALL
Unlock and invalidate all the D-Cache lines.
__STATIC_FORCEINLINE void MUnlockDCacheLine(unsigned long addr)
Unlock one D-Cache line specified by address in M-Mode.
__STATIC_FORCEINLINE void UFlushDCacheLine(unsigned long addr)
Flush one D-Cache line specified by address in U-Mode.
__STATIC_FORCEINLINE void MUnlockDCacheLines(unsigned long addr, unsigned long cnt)
Unlock several D-Cache lines specified by address in M-Mode.
@ CCM_DC_WBINVAL
Unlock, flush and invalidate the specific D-Cache line specified by CSR CCM_XBEGINADDR.
__STATIC_FORCEINLINE void SInvalICacheLine(unsigned long addr)
Invalidate one I-Cache line specified by address in S-Mode.
__STATIC_FORCEINLINE void EnableICache(void)
Enable ICache.
@ CCM_DC_INVAL
Unlock and invalidate D-Cache line specified by CSR CCM_XBEGINADDR.
__STATIC_FORCEINLINE void SUnlockICacheLines(unsigned long addr, unsigned long cnt)
Unlock several I-Cache lines specified by address in S-Mode.
__STATIC_FORCEINLINE unsigned long SLockDCacheLine(unsigned long addr)
Lock one D-Cache line specified by address in S-Mode.
__STATIC_FORCEINLINE void SInvalICacheLines(unsigned long addr, unsigned long cnt)
Invalidate several I-Cache lines specified by address in S-Mode.
#define MCACHE_CTL_IC_ECC_EN
__STATIC_FORCEINLINE int32_t GetDCacheInfo(CacheInfo_Type *info)
Get D-Cache Information.
@ CCM_OP_EXCEED_ERR
Exceed the the number of lockable ways(N-Way I/D-Cache, lockable is N-1)
__STATIC_FORCEINLINE void SInvalDCache(void)
Invalidate all D-Cache lines in S-Mode.
__STATIC_FORCEINLINE unsigned long MLockICacheLines(unsigned long addr, unsigned long cnt)
Lock several I-Cache lines specified by address in M-Mode.
#define CSR_CCM_UBEGINADDR
__STATIC_FORCEINLINE void UInvalDCacheLines(unsigned long addr, unsigned long cnt)
Invalidate several D-Cache lines specified by address in U-Mode.
__STATIC_FORCEINLINE int32_t ICachePresent(void)
Check ICache Unit Present or Not.
rv_csr_t lsize
D-Cache line size.
@ CCM_IC_UNLOCK
Unlock the specific I-Cache line specified by CSR CCM_XBEGINADDR.
@ CCM_IC_LOCK
Lock the specific I-Cache line specified by CSR CCM_XBEGINADDR.