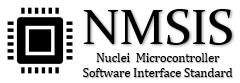 |
NMSIS-Core
Version 1.3.1
NMSIS-Core support for Nuclei processor-based devices
|
19 #ifndef __CORE_FEATURE_BASE__
20 #define __CORE_FEATURE_BASE__
37 #include "nmsis_compiler.h"
48 #define __RISCV_XLEN 32
50 #define __RISCV_XLEN __riscv_xlen
94 #if defined(__RISCV_XLEN) && __RISCV_XLEN == 64
110 #if defined(__RISCV_XLEN) && __RISCV_XLEN == 64
152 #if defined(__RISCV_XLEN) && __RISCV_XLEN == 64
173 #if defined(__RISCV_XLEN) && __RISCV_XLEN == 64
191 #if defined(__RISCV_XLEN) && __RISCV_XLEN == 64
208 #if defined(__RISCV_XLEN) && __RISCV_XLEN == 64
291 #if defined(__RISCV_XLEN) && __RISCV_XLEN == 64
496 #define __RV_CSR_SWAP(csr, val) \
498 rv_csr_t __v = (unsigned long)(val); \
499 __ASM volatile("csrrw %0, " STRINGIFY(csr) ", %1" \
514 #define __RV_CSR_READ(csr) \
517 __ASM volatile("csrr %0, " STRINGIFY(csr) \
532 #define __RV_CSR_WRITE(csr, val) \
534 rv_csr_t __v = (rv_csr_t)(val); \
535 __ASM volatile("csrw " STRINGIFY(csr) ", %0" \
551 #define __RV_CSR_READ_SET(csr, val) \
553 rv_csr_t __v = (rv_csr_t)(val); \
554 __ASM volatile("csrrs %0, " STRINGIFY(csr) ", %1" \
569 #define __RV_CSR_SET(csr, val) \
571 rv_csr_t __v = (rv_csr_t)(val); \
572 __ASM volatile("csrs " STRINGIFY(csr) ", %0" \
588 #define __RV_CSR_READ_CLEAR(csr, val) \
590 rv_csr_t __v = (rv_csr_t)(val); \
591 __ASM volatile("csrrc %0, " STRINGIFY(csr) ", %1" \
606 #define __RV_CSR_CLEAR(csr, val) \
608 rv_csr_t __v = (rv_csr_t)(val); \
609 __ASM volatile("csrc " STRINGIFY(csr) ", %0" \
616 #include <intrinsics.h>
618 #define __RV_CSR_SWAP __write_csr
619 #define __RV_CSR_READ __read_csr
620 #define __RV_CSR_WRITE __write_csr
621 #define __RV_CSR_READ_SET __set_bits_csr
622 #define __RV_CSR_SET __set_bits_csr
623 #define __RV_CSR_READ_CLEAR __clear_bits_csr
624 #define __RV_CSR_CLEAR __clear_bits_csr
640 unsigned long val = 0;
655 __ASM volatile(
"mv sp, %0" ::
"r"(stack));
657 __ASM volatile(
"mret");
932 #if __RISCV_XLEN == 32
933 volatile uint32_t high0, low, high;
942 full = (((uint64_t)high) << 32) | low;
944 #elif __RISCV_XLEN == 64
946 #else // TODO Need cover for XLEN=128 case in future
958 #if __RISCV_XLEN == 32
962 #elif __RISCV_XLEN == 64
964 #else // TODO Need cover for XLEN=128 case in future
976 #if __RISCV_XLEN == 32
977 volatile uint32_t high0, low, high;
986 full = (((uint64_t)high) << 32) | low;
988 #elif __RISCV_XLEN == 64
990 #else // TODO Need cover for XLEN=128 case in future
1002 #if __RISCV_XLEN == 32
1006 #elif __RISCV_XLEN == 64
1008 #else // TODO Need cover for XLEN=128 case in future
1021 #if __RISCV_XLEN == 32
1022 volatile uint32_t high0, low, high;
1028 if (high0 != high) {
1031 full = (((uint64_t)high) << 32) | low;
1033 #elif __RISCV_XLEN == 64
1035 #else // TODO Need cover for XLEN=128 case in future
1066 #ifdef __HARTID_OFFSET
1111 __ASM volatile(
"nop");
1126 __ASM volatile(
"wfi");
1139 __ASM volatile(
"wfi");
1152 __ASM volatile(
"ebreak");
1163 __ASM volatile(
"ecall");
1172 } WFI_SleepMode_Type;
1402 #if __RISCV_XLEN == 32
1491 #elif __RISCV_XLEN == 64
1538 #if __RISCV_XLEN == 32
1539 volatile uint32_t high0, low, high;
1549 full = (((uint64_t)high) << 32) | low;
return full;
1554 full = (((uint64_t)high) << 32) | low;
return full;
1559 full = (((uint64_t)high) << 32) | low;
return full;
1564 full = (((uint64_t)high) << 32) | low;
return full;
1569 full = (((uint64_t)high) << 32) | low;
return full;
1574 full = (((uint64_t)high) << 32) | low;
return full;
1579 full = (((uint64_t)high) << 32) | low;
return full;
1584 full = (((uint64_t)high) << 32) | low;
return full;
1589 full = (((uint64_t)high) << 32) | low;
return full;
1594 full = (((uint64_t)high) << 32) | low;
return full;
1599 full = (((uint64_t)high) << 32) | low;
return full;
1604 full = (((uint64_t)high) << 32) | low;
return full;
1609 full = (((uint64_t)high) << 32) | low;
return full;
1614 full = (((uint64_t)high) << 32) | low;
return full;
1619 full = (((uint64_t)high) << 32) | low;
return full;
1624 full = (((uint64_t)high) << 32) | low;
return full;
1629 full = (((uint64_t)high) << 32) | low;
return full;
1634 full = (((uint64_t)high) << 32) | low;
return full;
1639 full = (((uint64_t)high) << 32) | low;
return full;
1644 full = (((uint64_t)high) << 32) | low;
return full;
1649 full = (((uint64_t)high) << 32) | low;
return full;
1654 full = (((uint64_t)high) << 32) | low;
return full;
1659 full = (((uint64_t)high) << 32) | low;
return full;
1664 full = (((uint64_t)high) << 32) | low;
return full;
1669 full = (((uint64_t)high) << 32) | low;
return full;
1674 full = (((uint64_t)high) << 32) | low;
return full;
1679 full = (((uint64_t)high) << 32) | low;
return full;
1684 full = (((uint64_t)high) << 32) | low;
return full;
1689 full = (((uint64_t)high) << 32) | low;
return full;
1691 #elif __RISCV_XLEN == 64
1754 #define __FENCE(p, s) __ASM volatile ("fence " #p "," #s : : : "memory")
1764 __ASM volatile(
"fence.i");
1768 #define __RWMB() __FENCE(iorw,iorw)
1771 #define __RMB() __FENCE(ir,ir)
1774 #define __WMB() __FENCE(ow,ow)
1777 #define __SMP_RWMB() __FENCE(rw,rw)
1780 #define __SMP_RMB() __FENCE(r,r)
1783 #define __SMP_WMB() __FENCE(w,w)
1786 #define __CPU_RELAX() __ASM volatile ("" : : : "memory")
1800 __ASM volatile (
"lb %0, 0(%1)" :
"=r" (result) :
"r" (addr));
1814 __ASM volatile (
"lh %0, 0(%1)" :
"=r" (result) :
"r" (addr));
1828 __ASM volatile (
"lw %0, 0(%1)" :
"=r" (result) :
"r" (addr));
1832 #if __RISCV_XLEN != 32
1843 __ASM volatile (
"ld %0, 0(%1)" :
"=r" (result) :
"r" (addr));
1856 __ASM volatile (
"sb %0, 0(%1)" : :
"r" (val),
"r" (addr));
1867 __ASM volatile (
"sh %0, 0(%1)" : :
"r" (val),
"r" (addr));
1878 __ASM volatile (
"sw %0, 0(%1)" : :
"r" (val),
"r" (addr));
1881 #if __RISCV_XLEN != 32
1890 __ASM volatile (
"sd %0, 0(%1)" : :
"r" (val),
"r" (addr));
1911 "0: lr.w %0, %2 \n" \
1912 " bne %0, %z3, 1f \n" \
1913 " sc.w %1, %z4, %2 \n" \
1916 :
"=&r"(result),
"=&r"(rc),
"+A"(*addr) \
1917 :
"r"(oldval),
"r"(newval) \
1933 __ASM volatile (
"amoswap.w %0, %2, %1" : \
1934 "=r"(result),
"+A"(*addr) :
"r"(newval) :
"memory");
1949 __ASM volatile (
"amoadd.w %0, %2, %1" : \
1950 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
1965 __ASM volatile (
"amoand.w %0, %2, %1" : \
1966 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
1981 __ASM volatile (
"amoor.w %0, %2, %1" : \
1982 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
1997 __ASM volatile (
"amoxor.w %0, %2, %1" : \
1998 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2013 __ASM volatile (
"amomaxu.w %0, %2, %1" : \
2014 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2029 __ASM volatile (
"amomax.w %0, %2, %1" : \
2030 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2045 __ASM volatile (
"amominu.w %0, %2, %1" : \
2046 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2061 __ASM volatile (
"amomin.w %0, %2, %1" : \
2062 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2066 #if __RISCV_XLEN == 64
2078 __STATIC_FORCEINLINE uint64_t __CAS_D(
volatile uint64_t *addr, uint64_t oldval, uint64_t newval)
2084 "0: lr.d %0, %2 \n" \
2085 " bne %0, %z3, 1f \n" \
2086 " sc.d %1, %z4, %2 \n" \
2089 :
"=&r"(result),
"=&r"(rc),
"+A"(*addr) \
2090 :
"r"(oldval),
"r"(newval) \
2106 __ASM volatile (
"amoswap.d %0, %2, %1" : \
2107 "=r"(result),
"+A"(*addr) :
"r"(newval) :
"memory");
2122 __ASM volatile (
"amoadd.d %0, %2, %1" : \
2123 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2138 __ASM volatile (
"amoand.d %0, %2, %1" : \
2139 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2154 __ASM volatile (
"amoor.d %0, %2, %1" : \
2155 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2170 __ASM volatile (
"amoxor.d %0, %2, %1" : \
2171 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2186 __ASM volatile (
"amomaxu.d %0, %2, %1" : \
2187 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2202 __ASM volatile (
"amomax.d %0, %2, %1" : \
2203 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2218 __ASM volatile (
"amominu.d %0, %2, %1" : \
2219 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
2234 __ASM volatile (
"amomin.d %0, %2, %1" : \
2235 "=r"(result),
"+A"(*addr) :
"r"(value) :
"memory");
rv_csr_t safety_mecha
Indicate Core's safety mechanism.
rv_csr_t d
Type used for csr data access.
rv_csr_t mpp2
bit: 9..10 privilede mode of second level NMI/exception nestting
#define CSR_MHPMCOUNTER15H
rv_csr_t dc_ecc_chk_en
D-Cache check ECC codes enable.
rv_csr_t c
bit: 2 Compressed extension
__STATIC_FORCEINLINE void __enable_ext_irq_s(void)
Enable External IRQ Interrupts in supervisor mode.
rv_csr_t _reserved0
Reserved 0.
__STATIC_FORCEINLINE void __disable_sw_irq(void)
Disable software IRQ Interrupts.
rv_csr_t ppi_bpa
PPI base address.
rv_csr_t _reserved1
Reserved 0.
rv_csr_t ldspec_en
bit: 12 enable load speculative goes to mem interface
__STATIC_FORCEINLINE uint32_t __AMOMINU_W(volatile uint32_t *addr, uint32_t value)
Atomic unsigned MIN with 32bit value.
__STATIC_FORCEINLINE unsigned long __get_hart_index(void)
Get hart index of current cluster.
#define CSR_MHPMCOUNTER16
WFI_SleepMode
WFI Sleep Mode enumeration.
rv_csr_t d
Type used for csr data access.
rv_csr_t _reserved0
Reserved 0.
rv_csr_t addr
bit: 6..31 mtvec address
rv_csr_t d
bit: 3 Double-precision floating-point extension
rv_csr_t u
bit: 20 User mode implemented
rv_csr_t sramid
The ID of RAM that has 1bit ECC error, software can clear these bits.
rv_csr_t ic_en
I-Cache enable.
#define CSR_MHPMCOUNTER22H
rv_csr_t _reserved1
Reserved 0.
rv_csr_t d
Type used for csr data access.
rv_csr_t dlm_rwecc
Control mecc_code write to dlm, simulate error injection.
__STATIC_FORCEINLINE void __enable_mhpm_counters(unsigned long mask)
Enable hardware performance counters with mask.
rv_csr_t ppi_size
PPI size, need to be 2^n size.
#define CSR_MHPMCOUNTER20H
#define CSR_MHPMCOUNTER23H
rv_csr_t dc_ecc_en
D-Cache ECC enable.
rv_csr_t s
bit: 18 Supervisor mode implemented
__STATIC_FORCEINLINE void __disable_mcycle_counter(void)
Disable MCYCLE counter.
__STATIC_FORCEINLINE void __enable_timer_irq(void)
Enable Timer IRQ Interrupts.
__STATIC_FORCEINLINE void __disable_timer_irq(void)
Disable Timer IRQ Interrupts.
rv_csr_t dcache
DCache present.
rv_csr_t typ
bit: 6..7 current trap type
#define CSR_MHPMCOUNTER14
rv_csr_t lsize
I-Cache line size.
#define CSR_MHPMCOUNTER17H
#define CSR_MHPMCOUNTER21
rv_csr_t d
Type used for csr data access.
rv_csr_t _reserved0
Reserved.
rv_csr_t _reserved0
bit: 0 Reserved
rv_csr_t ic_ecc_excp_en
I-Cache 2bit ECC error exception enable.
__STATIC_FORCEINLINE void __enable_irq_s(void)
Enable IRQ Interrupts in supervisor mode.
rv_csr_t set
Main TLB sets per way.
__STATIC_FORCEINLINE void __enable_sw_irq(void)
Enable software IRQ Interrupts.
__STATIC_FORCEINLINE void __NOP(void)
NOP Instruction.
#define __RV_CSR_CLEAR(csr, val)
CSR operation Macro for csrc instruction.
__STATIC_FORCEINLINE void __disable_sw_irq_s(void)
Disable software IRQ Interrupts in supervisor mode.
rv_csr_t mpie2
bit: 8 interrupt enable flag of second level NMI/exception nestting
Union type to access MFIOCFG_INFO CSR register.
rv_csr_t _reserved0
Reserved.
Union type to access MCACHE_CTL CSR register.
rv_csr_t ilm_en
ILM enable.
rv_csr_t xs
bit: XS status flag
rv_csr_t dc_en
DCache enable.
rv_csr_t _reserved2
bit: 16..31 Reserved
__STATIC_FORCEINLINE unsigned long __get_hpm_event(unsigned long idx)
Get event for selected high performance monitor event.
rv_csr_t mode
bit: 0..5 interrupt mode control
rv_csr_t _reserved1
bit: 26..24 Reserved
__STATIC_FORCEINLINE void __ECALL(void)
Environment Call Instruction.
#define CSR_MHPMCOUNTER22
#define CSR_MHPMCOUNTER31
rv_csr_t misalign
bit: 6 misaligned access support flag
Union type to access MECC_CODE CSR register.
rv_csr_t vpu_degree
Indicate the VPU degree of parallel.
rv_csr_t ilm_ecc_chk_en
ILM check ECC codes enable.
Union type to access MCOUNTINHIBIT CSR register.
rv_csr_t core_buserr
bit: 8 core bus error exception or interrupt
Union type to access MICFG_INFO CSR register.
rv_csr_t _reserved0
bit: 1 Reserved
#define CSR_MHPMCOUNTER27H
rv_csr_t _reserved3
bit: Reserved
#define CSR_MHPMCOUNTER13
rv_csr_t vnice
VNICE present.
__STATIC_FORCEINLINE int32_t __AMOAND_W(volatile int32_t *addr, int32_t value)
Atomic And with 32bit value.
rv_csr_t ptyp1
bit: 6..7 NMI/exception type of before first nestting
rv_csr_t _reserved1
Reserved.
rv_csr_t sijump_en
bit: 11 SIJUMP mode of trace
#define CSR_MHPMCOUNTER10H
Union type to access MCFG_INFO CSR register.
#define CSR_MHPMCOUNTER14H
rv_csr_t spie
bit: 3 Supervisor Privilede mode interrupt enable flag
rv_csr_t d
Type used for csr data access.
rv_csr_t clic
CLIC present.
__STATIC_FORCEINLINE void __disable_ext_irq_s(void)
Disable External IRQ Interrupts in supervisor mode.
#define CSR_MHPMCOUNTER13H
rv_csr_t i_size
ITLB size.
rv_csr_t mpp1
bit: 1..2 privilede mode of fisrt level NMI/exception nestting
__STATIC_FORCEINLINE int32_t __AMOXOR_W(volatile int32_t *addr, int32_t value)
Atomic XOR with 32bit value.
rv_csr_t _reserved1
bit: 2 Reserved
rv_csr_t fio_size
FIO size, need to be 2^n size.
__STATIC_FORCEINLINE int32_t __AMOMAX_W(volatile int32_t *addr, int32_t value)
Atomic signed MAX with 32bit value.
Union type to access MDCFG_INFO CSR register.
Union type to access MCAUSE CSR register.
rv_csr_t d
Type used for csr data access.
rv_csr_t mpil
bit: 23..16 Previous interrupt level
rv_csr_t ptyp
bit: 8..9 previous trap type
Union type to access MISA CSR register.
#define CSR_MHPMCOUNTER20
__STATIC_FORCEINLINE void __set_wfi_sleepmode(WFI_SleepMode_Type mode)
Set Sleep mode of WFI.
#define CSR_MHPMCOUNTER8H
rv_csr_t _reserved1
bit: 4..5 Reserved
rv_csr_t t
bit: 19 Tentatively reserved for Transactional Memory extension
rv_csr_t ptyp2
bit: 14..15 NMI/exception type of before second nestting
rv_csr_t mxl
bit: 30..31 Machine XLEN
rv_csr_t _reserved1
bit: 11..13 Reserved
#define __RV_CSR_WRITE(csr, val)
CSR operation Macro for csrw instruction.
rv_csr_t f
bit: 5 Single-precision floating-point extension
Union type to access MDLM_CTL CSR register.
rv_csr_t _reserved1
Reserved 0.
__STATIC_FORCEINLINE uint32_t __get_core_irq_pending(uint32_t irq)
Get Core IRQ Interrupt Pending status.
rv_csr_t ilm_bpa
ILM base address.
rv_csr_t d
Type used for csr data access.
rv_csr_t j
bit: 9 Tentatively reserved for Dynamically Translated Languages extension
__STATIC_FORCEINLINE void __set_hpm_event(unsigned long idx, unsigned long event)
Set event for selected high performance monitor event.
rv_csr_t lm_xonly
ILM Execute only permission.
__STATIC_FORCEINLINE uint16_t __LH(volatile void *addr)
Load 16bit value from address (16 bit)
rv_csr_t g
bit: 6 Additional standard extensions present
__STATIC_FORCEINLINE uint32_t __LW(volatile void *addr)
Load 32bit value from address (32 bit)
CSR_MMISCCTRL_Type CSR_MMISCCTL_Type
rv_csr_t dlm_en
DLM enable.
__STATIC_FORCEINLINE void __enable_timer_irq_s(void)
Enable Timer IRQ Interrupts in supervisor mode.
__STATIC_FORCEINLINE void __clear_core_irq_pending(uint32_t irq)
Clear Core IRQ Interrupt Pending status.
rv_csr_t dlm_ecc_excp_en
DLM ECC exception enable.
rv_csr_t mpie1
bit: 0 interrupt enable flag of fisrt level NMI/exception nestting
rv_csr_t d
Type used for csr data access.
rv_csr_t fs
bit: FS status flag
#define CSR_MHPMCOUNTER17
rv_csr_t lm_ecc
DLM ECC present.
rv_csr_t _reserved2
bit: 13 Reserved
__STATIC_FORCEINLINE unsigned long __get_hart_id(void)
Get hart id of current cluster.
rv_csr_t mpie
bit: 27 Interrupt enable flag before enter interrupt
rv_csr_t d
Type used for csr data access.
Union type to access MSUBM CSR register.
#define CSR_MHPMCOUNTER19H
__STATIC_FORCEINLINE void __disable_irq_s(void)
Disable IRQ Interrupts in supervisor mode.
rv_csr_t cy
bit: 0 1 means disable mcycle counter
rv_csr_t l
bit: 11 Tentatively reserved for Decimal Floating-Point extension
rv_csr_t ic_ecc_en
I-Cache ECC enable.
Union type to access MTVEC CSR register.
rv_csr_t dlm_ecc_en
DLM ECC eanble.
__STATIC_FORCEINLINE void __set_rv_cycle(uint64_t cycle)
Set whole 64 bits value of mcycle counter.
rv_csr_t d_size
DTLB size.
__STATIC_FORCEINLINE void __disable_all_counter(void)
Disable all MCYCLE & MINSTRET & MHPMCOUNTER counter.
Union type to access MMISC_CTRL CSR register.
#define CSR_MHPMCOUNTER18H
__STATIC_FORCEINLINE void __SB(volatile void *addr, uint8_t val)
Write 8bit value to address (8 bit)
rv_csr_t icache
ICache present.
__STATIC_FORCEINLINE void __disable_mhpm_counters(unsigned long mask)
Disable hardware performance counters with mask.
rv_csr_t sum
bit: Supervisor Mode load and store protection
rv_csr_t etrace
Etrace present.
#define CSR_MHPMCOUNTER24
rv_csr_t h
bit: 7 Hypervisor extension
rv_csr_t b
bit: 1 Tentatively reserved for Bit-Manipulation extension
rv_csr_t lm_size
DLM size, need to be 2^n size.
__STATIC_FORCEINLINE void __disable_timer_irq_s(void)
Disable Timer IRQ Interrupts in supervisor mode.
rv_csr_t ic_rwtecc
Control I-Cache Tag Ram ECC code injection.
__STATIC_FORCEINLINE void __enable_ext_irq(void)
Enable External IRQ Interrupts.
__STATIC_FORCEINLINE uint64_t __get_rv_time(void)
Read whole 64 bits value of real-time clock.
rv_csr_t ic_scpd_mod
Scratchpad mode, 0: Scratchpad as ICache Data RAM, 1: Scratchpad as ILM SRAM.
rv_csr_t minhv
bit: 30 Machine interrupt vector table
rv_csr_t lm_ecc
ILM ECC present.
rv_csr_t _reserved1
bit: 10 Reserved
Union type to access MTLBCFG_INFO CSR register.
rv_csr_t zcmt_zcmp
bit: 7 Zc Ext uses the cfdsp of D Ext’s encoding or not
#define CSR_MHPMCOUNTER30H
__STATIC_FORCEINLINE uint32_t __AMOMAXU_W(volatile uint32_t *addr, uint32_t value)
Atomic unsigned MAX with 32bit value.
__STATIC_FORCEINLINE void __enable_core_irq(uint32_t irq)
Enable Core IRQ Interrupt.
__STATIC_FORCEINLINE void __WFI(void)
Wait For Interrupt.
rv_csr_t code
Used to inject ECC check code.
rv_csr_t mpie
bit: mirror of MIE flag
rv_csr_t zc_xlcz
Zc and xlcz extension present.
rv_csr_t dsp_n2
DSP N2 present.
rv_csr_t d
Type used for csr data access.
rv_csr_t iregion
IREGION present.
__STATIC_FORCEINLINE void __enable_mcycle_counter(void)
Enable MCYCLE counter.
__STATIC_FORCEINLINE void __disable_irq(void)
Disable IRQ Interrupts.
rv_csr_t ilm_rwecc
Control mecc_code write to ilm, simulate error injection.
__STATIC_FORCEINLINE void __enable_mhpm_counter(unsigned long idx)
Enable selected hardware performance monitor counter.
rv_csr_t _reserved0
Reserved.
@ WFI_SHALLOW_SLEEP
Shallow sleep mode, the core_clk will poweroff.
rv_csr_t ilm_ecc_en
ILM ECC eanble.
Union type to access MECC_LOCK CSR register.
rv_csr_t ic_pf_en
I-Cache prefetch enable.
__STATIC_FORCEINLINE uint32_t __CAS_W(volatile uint32_t *addr, uint32_t oldval, uint32_t newval)
Compare and Swap 32bit value using LR and SC.
__STATIC_FORCEINLINE uint64_t __get_rv_cycle(void)
Read whole 64 bits value of mcycle counter.
#define CSR_MHPMCOUNTER16H
rv_csr_t _reserved2
Reserved 0.
__STATIC_FORCEINLINE void __switch_mode(uint8_t mode, uintptr_t stack, void(*entry_point)(void))
switch privilege from machine mode to others.
__STATIC_FORCEINLINE int32_t __AMOOR_W(volatile int32_t *addr, int32_t value)
Atomic OR with 32bit value.
rv_csr_t d
Type used for csr data access.
rv_csr_t ecc_lock
RW permission, ECC Lock configure.
rv_csr_t set
I-Cache sets per way.
__STATIC_FORCEINLINE void __SH(volatile void *addr, uint16_t val)
Write 16bit value to address (16 bit)
rv_csr_t exccode
bit: 11..0 exception or interrupt code
#define CSR_MHPMCOUNTER4H
#define CSR_MHPMCOUNTER12
#define CSR_MHPMCOUNTER28H
#define CSR_MHPMCOUNTER25
rv_csr_t ir
bit: 2 1 means disable minstret counter
rv_csr_t way
Main TLB ways.
#define CSR_MHPMCOUNTER9H
rv_csr_t mpp
bit: mirror of Privilege Mode
#define CSR_MHPMCOUNTER11
#define CSR_MHPMCOUNTER7H
__STATIC_FORCEINLINE void __enable_core_irq_s(uint32_t irq)
Enable Core IRQ Interrupt in supervisor mode.
rv_csr_t dlm_bpa
DLM base address.
__STATIC_FORCEINLINE void __enable_irq(void)
Enable IRQ Interrupts.
#define CSR_MHPMCOUNTER27
#define __STATIC_FORCEINLINE
Define a static function that should be always inlined by the compiler.
rv_csr_t dc_rwtecc
Control D-Cache Tag Ram ECC code injection.
#define CSR_MCOUNTINHIBIT
#define CSR_MHPMCOUNTER5H
__STATIC_FORCEINLINE uint32_t __get_core_irq_pending_s(uint32_t irq)
Get Core IRQ Interrupt Pending status in supervisor mode.
__STATIC_FORCEINLINE unsigned long __get_cluster_id(void)
Get cluster id of current cluster.
rv_csr_t sec_mode
Smwg extension present.
rv_csr_t dc_ecc_excp_en
D-Cache 2bit ECC error exception enable.
rv_csr_t _reserved0
bit: 0..2 Reserved
Union type to access MSTATUS CSR register.
rv_csr_t d
Type used for csr data access.
rv_csr_t d
Type used for csr data access.
__STATIC_FORCEINLINE void __TXEVT(void)
Send TX Event.
__STATIC_FORCEINLINE void __EBREAK(void)
Breakpoint Instruction.
#define CSR_MHPMCOUNTER29H
#define CSR_MHPMCOUNTER12H
__STATIC_FORCEINLINE void __enable_sw_irq_s(void)
Enable software IRQ Interrupts in supervisor mode.
rv_csr_t dc_rwdecc
Control D-Cache Data Ram ECC code injection.
__STATIC_FORCEINLINE uint32_t __AMOSWAP_W(volatile uint32_t *addr, uint32_t newval)
Atomic Swap 32bit value into memory.
Union type to access MDCAUSE CSR register.
rv_csr_t set
D-Cache sets per way.
#define __RV_CSR_READ(csr)
CSR operation Macro for csrr instruction.
rv_csr_t bpu
bit: 3 dynamic prediction enable flag
rv_csr_t ic_ecc_chk_en
I-Cache check ECC codes enable.
rv_csr_t plic
PLIC present.
__STATIC_FORCEINLINE void __set_rv_instret(uint64_t instret)
Set whole 64 bits value of machine instruction-retired counter.
rv_csr_t _reserved0
Reserved.
rv_csr_t lsize
Main TLB line size.
#define CSR_MHPMCOUNTER24H
rv_csr_t mdcause
bit: 0..2 More detailed exception information as MCAUSE supplement
__STATIC_FORCEINLINE unsigned long __get_hpm_counter(unsigned long idx)
Get value of selected high performance monitor couner.
rv_csr_t _reserved4
bit: Reserved
#define CSR_MHPMCOUNTER3H
__STATIC_FORCEINLINE void __disable_minstret_counter(void)
Disable MINSTRET counter.
#define CSR_MHPMCOUNTER15
rv_csr_t a
bit: 0 Atomic extension
rv_csr_t w
Type used for csr data access.
#define CSR_MHPMCOUNTER19
__STATIC_FORCEINLINE void __enable_all_counter(void)
Enable all MCYCLE & MINSTRET & MHPMCOUNTER counter.
rv_csr_t mprv
bit: Machine mode PMP
#define CSR_MHPMCOUNTER11H
__STATIC_FORCEINLINE void __enable_minstret_counter(void)
Enable MINSTRET counter.
#define CSR_MHPMCOUNTER23
#define CSR_MHPMCOUNTER26H
rv_csr_t _reserved6
bit: 19..30 Reserved
rv_csr_t ecc
Main TLB supports ECC or not.
rv_csr_t p
bit: 15 Tentatively reserved for Packed-SIMD extension
#define __RV_CSR_SET(csr, val)
CSR operation Macro for csrs instruction.
#define CSR_MHPMCOUNTER31H
#define CSR_MHPMCOUNTER21H
rv_csr_t dsp_n3
DSP N3 present.
__STATIC_FORCEINLINE void __WFE(void)
Wait For Event.
rv_csr_t d
Type used for csr data access.
rv_csr_t _reserved4
bit: 22 Reserved
#define CSR_MHPMCOUNTER28
rv_csr_t m
bit: 12 Integer Multiply/Divide extension
rv_csr_t _reserved0
bit: 15..12 Reserved
#define CSR_MHPMCOUNTER29
rv_csr_t _reserved5
bit: 24..29 Reserved
rv_csr_t d
Type used for csr data access.
rv_csr_t ic_cancel_en
I-Cache change flow canceling enable control.
__STATIC_FORCEINLINE int32_t __AMOADD_W(volatile int32_t *addr, int32_t value)
Atomic Add with 32bit value.
rv_csr_t fio_bpa
FIO base address.
__STATIC_FORCEINLINE uint8_t __LB(volatile void *addr)
Load 8bit value from address (8 bit)
rv_csr_t _reserved0
bit: 0..5 Reserved
#define CSR_MHPMCOUNTER30
rv_csr_t imreturn_en
bit: 10 IMRETURN mode of trace
rv_csr_t sd
bit: Dirty status for XS or FS
rv_csr_t _reserved2
bit: 14 Reserved
#define __RISCV_XLEN
Refer to the width of an integer register in bits(either 32 or 64)
rv_csr_t dlm_ecc_chk_en
DLM check ECC codes enable.
__STATIC_FORCEINLINE void __disable_ext_irq(void)
Disable External IRQ Interrupts.
rv_csr_t _reserved2
bit: 4 Reserved
rv_csr_t q
bit: 16 Quad-precision floating-point extension
rv_csr_t sie
bit: 1 supervisor interrupt enable flag
rv_csr_t ilm_ecc_excp_en
ILM ECC exception enable.
__STATIC_FORCEINLINE int32_t __AMOMIN_W(volatile int32_t *addr, int32_t value)
Atomic signed MIN with 32bit value.
__STATIC_FORCEINLINE void __disable_core_irq(uint32_t irq)
Disable Core IRQ Interrupt.
rv_csr_t _reserved1
bit: 10..31 Reserved
rv_csr_t _reserved3
bit: 15..XLEN-1 Reserved
__STATIC_FORCEINLINE void __set_medeleg(unsigned long mask)
Set exceptions delegation to S mode.
rv_csr_t _reserved1
bit: 3..31 Reserved
rv_csr_t d
Type used for csr data access.
rv_csr_t dsp_n1
DSP N1 present.
Union type to access MSAVESTATUS CSR register.
__STATIC_FORCEINLINE void __set_hpm_counter(unsigned long idx, uint64_t value)
Set value for selected high performance monitor counter.
rv_csr_t d
Type used for csr data access.
rv_csr_t v
bit: 21 Tentatively reserved for Vector extension
rv_csr_t d
Type used for csr data access.
__STATIC_FORCEINLINE void __SW(volatile void *addr, uint32_t val)
Write 32bit value to address (32 bit)
rv_csr_t interrupt
bit: 31 trap type.
__STATIC_FORCEINLINE void __disable_core_irq_s(uint32_t irq)
Disable Core IRQ Interrupt in supervisor mode.
rv_csr_t ramid
The ID of RAM that has 2bit ECC error, software can clear these bits.
Union type to access MPPICFG_INFO CSR register.
rv_csr_t _reserved0
bit: 3..XLEN-1 Reserved
#define CSR_MHPMCOUNTER25H
#define __ASM
Pass information from the compiler to the assembler.
__STATIC_FORCEINLINE uint64_t __get_rv_instret(void)
Read whole 64 bits value of machine instruction-retired counter.
@ WFI_DEEP_SLEEP
Deep sleep mode, the core_clk and core_ano_clk will poweroff.
rv_csr_t _reserved0
bit: 3..5 Reserved
rv_csr_t e
bit: 4 RV32E base ISA
Union type to access MILM_CTL CSR register.
__STATIC_FORCEINLINE void __FENCE_I(void)
Fence.i Instruction.
rv_csr_t lm_size
ILM size, need to be 2^n size.
unsigned long rv_csr_t
Type of Control and Status Register(CSR), depends on the XLEN defined in RISC-V.
rv_csr_t nice
NICE present.
#define CSR_MHPMCOUNTER10
#define CSR_MHPMCOUNTER18
rv_csr_t lsize
D-Cache line size.
rv_csr_t mpp
bit: 29..28 Privilede mode flag before enter interrupt
__STATIC_FORCEINLINE void __disable_mhpm_counter(unsigned long idx)
Disable selected hardware performance monitor counter.
__STATIC_FORCEINLINE void __clear_core_irq_pending_s(uint32_t irq)
Clear Core IRQ Interrupt Pending status in supervisor mode.
#define CSR_MHPMCOUNTER6H
rv_csr_t n
bit: 13 User-level interrupts supported
rv_csr_t _reserved0
Reserved 1.
#define CSR_MHPMCOUNTER26
rv_csr_t nmi_cause
bit: 9 mnvec control and nmi mcase exccode
rv_csr_t mie
bit: 3 Machine mode interrupt enable flag
rv_csr_t x
bit: 23 Non-standard extensions present
rv_csr_t ic_rwdecc
Control I-Cache Data Ram ECC code injection.
rv_csr_t i
bit: 8 RV32I/64I/128I base ISA
rv_csr_t _resreved3
bit: 17 Reserved
rv_csr_t dbg_sec
bit: 14 debug access mode